Python is a versatile, high-level programming language known for its readability, simplicity, and broad applicability. It was created by Guido van Rossum and first released in 1991. Python’s design philosophy emphasizes code readability and syntax that allows programmers to express concepts in fewer lines of code compared to other languages like C++ or Java.
Key Features of Python:
- Simple and Easy to Learn: Python’s syntax is clear and easy to understand, making it an ideal language for beginners.
- Interpreted Language: Python is an interpreted language, meaning that the code is executed line by line, which helps in debugging.
- Dynamically Typed: In Python, you don’t need to declare the type of a variable explicitly. The type is determined at runtime.
- Extensive Standard Library: Python has a rich set of libraries and frameworks that support a variety of applications, including web development, data analysis, machine learning, and more.
- Cross-Platform: Python is platform-independent, which means the same code can run on different operating systems like Windows, macOS, and Linux.
- Object-Oriented: Python supports object-oriented programming (OOP), which allows for concepts like inheritance, encapsulation, and polymorphism.
- Large Community and Ecosystem: Python has a large community of developers, which means extensive documentation, a wealth of tutorials, and a large selection of third-party packages.
Basic Syntax:
Here’s a simple example to demonstrate Python’s syntax:
# This is a comment in Python
print("Hello, World!") # This will print Hello, World! to the console
# Variables and types
x = 5 # Integer
y = 3.2 # Float
name = "John" # String
# Conditional statements
if x > y:
print(f"{x} is greater than {y}")
else:
print(f"{x} is not greater than {y}")
# Loops
for i in range(5): # Loops from 0 to 4
print(i)
# Functions
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
Applications of Python:
- Web Development: Frameworks like Django and Flask allow for robust web development.
- Data Science and Machine Learning: Libraries like Pandas, NumPy, SciPy, and TensorFlow are widely used.
- Automation: Python scripts can automate repetitive tasks.
- Game Development: Libraries like Pygame help in developing games.
- Networking: Python can be used to create networking applications using libraries like Twisted and Socket.
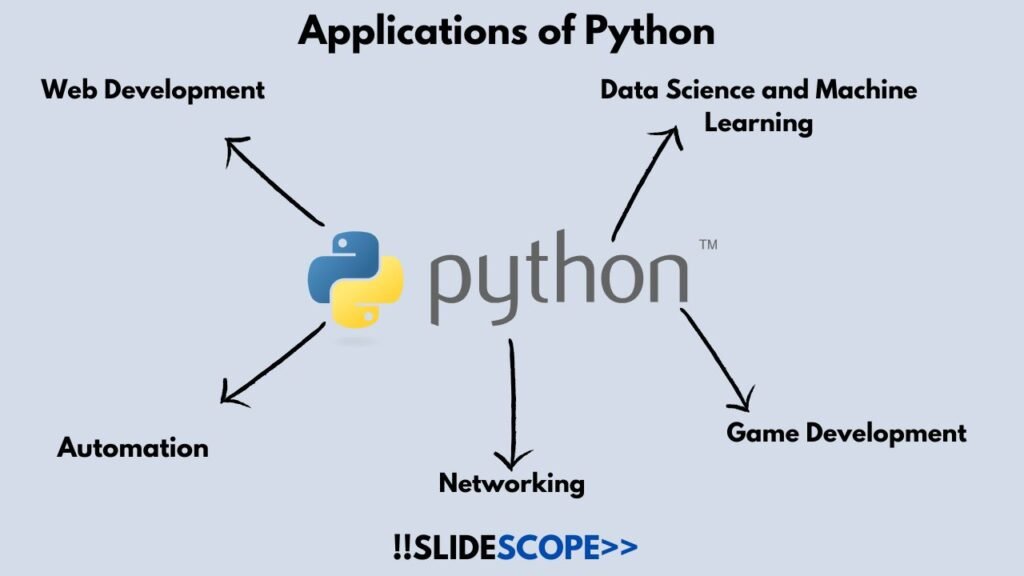
Getting Started:
To start programming in Python, you need to install Python from the official Python website. You can write Python code in any text editor, but using an Integrated Development Environment (IDE) like PyCharm, VS Code, or even Jupyter Notebooks can enhance productivity.
Would you like to dive deeper into any specific area of Python? – Learn Python at <a href=”https://slidescope.com”>Slidescope</a>